Maven divides execution into four nested hierarchies. From most-encompassing to most-specific, they are: Lifecycle, Phase, Plugin, and Goal.
Lifecycles, Phases, Plugins and Goals
Maven defines the concept of language-independent project build flows that model the steps that all software goes through during a compilation and deployment process.
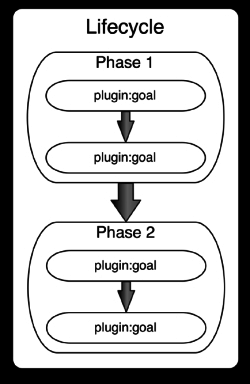
Lifecycles represent a well-recognized flow of steps (Phases) used in software assembly.
Each step in a lifecycle flow is called a phase. Zero or more plugin goals are bound to a phase.
A plugin is a logical grouping and distribution (often a single JAR) of related goals, such as JARing.
A goal, the most granular step in Maven, is a single executable task within a plugin. For example, discrete goals in the jar plugin include packaging the jar (jar:jar), signing the jar (jar:sign), and verifying the signature (jar:sign-verify).
Executing a Phase or Goal
At the command prompt, either a phase or a plugin goal can be requested. Multiple phases or goals can be specified and are separated by spaces.
If you ask Maven to run a specific plugin goal, then only that goal is run. This example runs two plugin goals: compilation
of code, then JARing the result, skipping over any intermediate steps.
mvn compile:compile jar:jar
Conversely, if you ask Maven to execute a phase, all phases and bound plugin goals up to that point in the lifecycle are also executed. This example requests the deploy lifecycle phase, which will also execute the verification, compilation, testing and packaging phases.
mvn deploy
Online and Offline
During a build, Maven attempts to download any uncached referenced artifacts and proceeds to cache them in the ~/.m2/repository directory on Unix, or in the %USERPROFILE%/.m2/repository directory on Windows.
To prepare for compiling offline, you can instruct Maven to download all referenced artifacts from the Internet via the command:
mvn dependency:go-offline
If all required artifacts and plugins have been cached in your local repository, you can instruct Maven to run in offline mode with a simple flag:
mvn <phase or goal> -o
Built-In Maven Lifecycles
Maven ships with three lifecycles; clean, default, and site. Many of the phases within these three lifecycles are bound to a sensible plugin goal.

The official lifecycle reference, which extensively lists all the default bindings, can be found at http://maven.apache.org/guides/introduction/introduction-to-the-lifecycle.html
The clean lifecycle is simplistic in nature. It deletes all generated and compiled artifacts in the output directory.
Clean Lifecycle |
Lifecycle Phase |
Purpose |
pre-clean |
|
clean |
Remove all generated and compiled artifacts in preperation for a fresh build. |
post-clean |
|
Default Lifecycle |
Lifecycle Phase |
Purpose |
validate |
Cross check that all elements necessary for the build are correct and present. |
initialize |
Set up and bootstrap the build process. |
generate-sources |
Generate dynamic source code |
process-sources |
Filter, sed and copy source code |
generate-resources |
Generate dynamic resources |
process-resources |
Filter, sed and copy resources files. |
compile |
Compile the primary or mixed language source files. |
process-classes |
Augment compiled classes, such as for code-coverage instrumentation. |
generate-test-sources |
Generate dynamic unit test source code. |
process-test-sources |
Filter, sed and copy unit test source code. |
generate-test-resources |
Generate dynamic unit test resources. |
process-test-resources |
Filter, sed and copy unit test resources. |
test-compile |
Compile unit test source files |
test |
Execute unit tests |
prepare-package |
Manipulate generated artifacts immediately prior to packaging. (Maven 2.1 and above) |
package |
Bundle the module or application into a distributable package (commonly, JAR, WAR, or EAR). |
pre-integration-test |
|
integration-test |
Execute tests that require connectivity to external resources or other components |
post-integration-test |
|
verify |
Inspect and cross-check the distribution package (JAR, WAR, EAR) for correctness. |
install |
Place the package in the user’s local Maven repository. |
deploy |
Upload the package to a remote Maven repository |
The site lifecycle generates a project information web site, and can deploy the artifacts to a specified web server or local path.
Site Lifecycle |
Lifecycle Phase |
Purpose |
pre-site |
Cross check that all elements necessary for the build are correct and present. |
site |
Generate an HTML web site containing project information and reports. |
post-site |
|
site-deploy |
Upload the generated website to a web server |
Default Goal
The default goal codifies the author’s intended usage of the build script. Only one goal or lifecycle can be set as the default. The most common default goal is install.
<project>
[...]
<build>
lt;defaultGoal>install</defaultGoal>
</build>
[...]
</project>
{{ parent.title || parent.header.title}}
{{ parent.tldr }}
{{ parent.linkDescription }}
{{ parent.urlSource.name }}